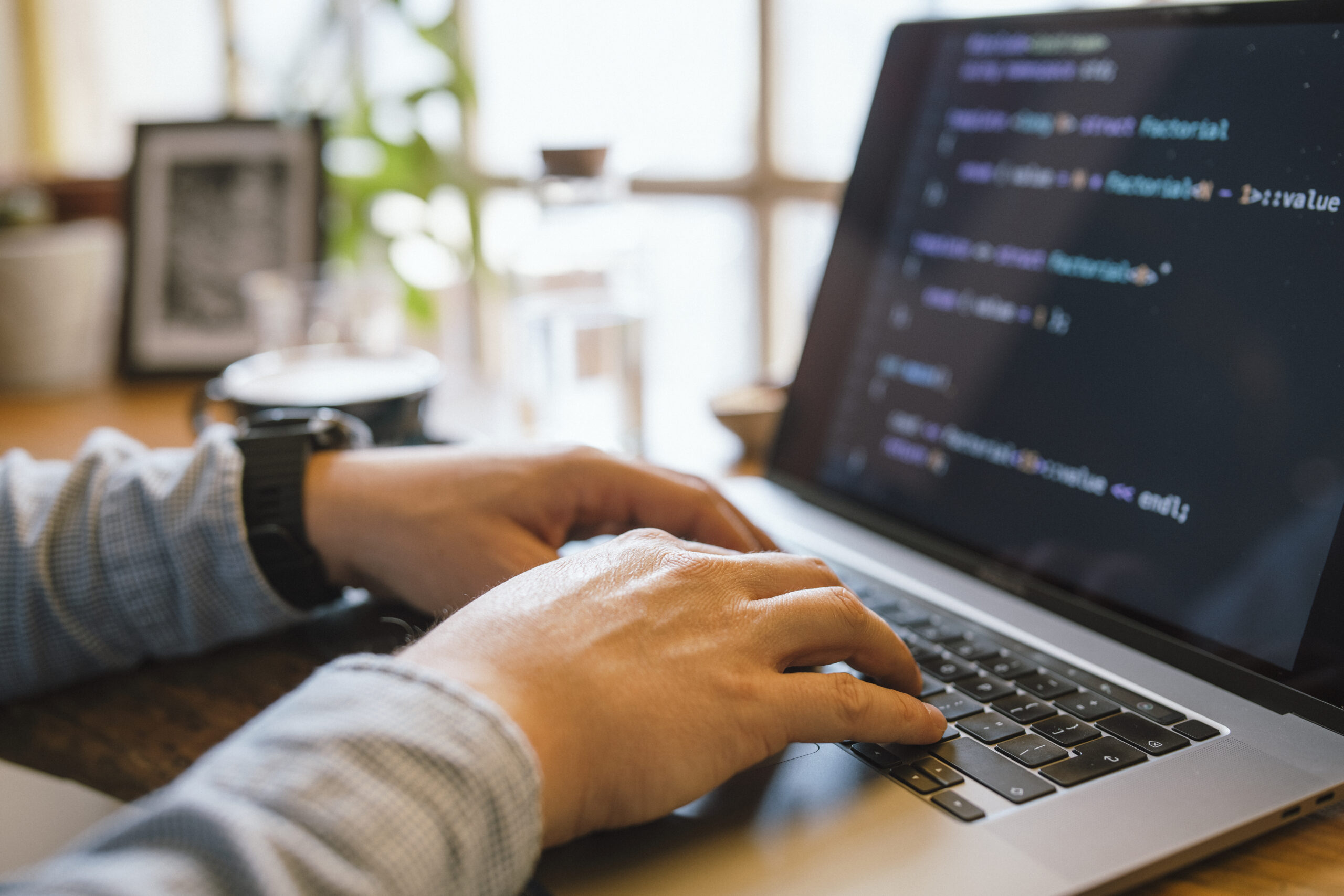
Debugging is Probably the most necessary — however usually overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems proficiently. No matter if you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve several hours of irritation and radically help your efficiency. Here's many procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of quickest means builders can elevate their debugging expertise is by mastering the resources they use every day. Though creating code is one Element of progress, being aware of how you can connect with it effectively all through execution is Similarly essential. Fashionable progress environments arrive equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these applications can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used the right way, they Allow you to notice specifically how your code behaves all through execution, which can be a must have for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch community requests, view authentic-time overall performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can turn annoying UI issues into manageable responsibilities.
For backend or program-level builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around managing procedures and memory management. Mastering these tools could have a steeper Discovering curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, become comfy with Model Command methods like Git to grasp code heritage, find the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment usually means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement ecosystem so that when issues arise, you’re not lost at midnight. The better you realize your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often ignored — steps in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a consistent ecosystem or circumstance the place the bug reliably appears. Without the need of reproducibility, correcting a bug results in being a match of likelihood, often bringing about wasted time and fragile code changes.
The initial step in reproducing a dilemma is collecting as much context as is possible. Request concerns like: What steps resulted in the issue? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the less difficult it becomes to isolate the precise circumstances underneath which the bug occurs.
As you’ve collected sufficient information and facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic exams that replicate the sting cases or condition transitions included. These tests not merely assist expose the situation but also avoid regressions Sooner or later.
Sometimes, The problem could be natural environment-certain — it would materialize only on particular running devices, browsers, or under specific configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're presently halfway to fixing it. Using a reproducible situation, You need to use your debugging equipment far more effectively, test possible fixes safely, and communicate more clearly with your workforce or consumers. It turns an abstract complaint right into a concrete obstacle — and that’s in which developers prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when something goes wrong. As opposed to seeing them as frustrating interruptions, builders really should understand to treat error messages as immediate communications within the process. They typically let you know exactly what transpired, the place it occurred, and sometimes even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in comprehensive. A lot of builders, especially when less than time strain, glance at the primary line and right away start generating assumptions. But deeper in the mistake stack or logs might lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — study and fully grasp them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it issue to a particular file and line selection? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable styles, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, As well as in All those situations, it’s very important to examine the context during which the mistake happened. Check associated log entries, input values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for general situations (like successful get started-ups), Alert for prospective problems that don’t crack the applying, ERROR for actual complications, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. Too much logging can obscure vital messages and slow down your technique. Concentrate on key gatherings, state variations, enter/output values, and critical final decision points inside your code.
Structure your log messages Obviously and consistently. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the end, wise logging is about stability and clarity. Which has a nicely-considered-out logging solution, you'll be able to decrease the time it takes to spot challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective fixing a thriller. This frame of mind can help stop working elaborate problems into manageable elements and stick to clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency concerns. Identical to a detective surveys against the Gustavo Woltmann coding law scene, obtain just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire yourself: What could be causing this conduct? Have any changes recently been built into the codebase? Has this challenge transpired prior to under identical situation? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and Enable the final results lead you closer to the reality.
Pay near interest to compact information. Bugs often cover within the the very least predicted places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely knowing it. Non permanent fixes could disguise the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for long run problems and support others realize your reasoning.
By imagining similar to a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing assessments is among the simplest ways to boost your debugging capabilities and Over-all development efficiency. Tests not just aid catch bugs early but in addition function a security Internet that offers you assurance when earning changes for your codebase. A nicely-tested application is easier to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which target particular person features or modules. These modest, isolated checks can immediately expose no matter if a certain piece of logic is Functioning as expected. When a exam fails, you straight away know wherever to glance, significantly lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Next, combine integration exams and finish-to-close assessments into your workflow. These aid make sure that various portions of your application work jointly easily. They’re notably beneficial for catching bugs that occur in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what ailments.
Creating checks also forces you to Imagine critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This amount of knowing The natural way prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the check fails continually, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the trouble—watching your display screen for several hours, seeking solution following Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, decrease aggravation, and often see the issue from a new perspective.
When you're too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you wrote just hours before. During this point out, your Mind turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or simply switching to a special task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also help reduce burnout, In particular in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You may quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
Briefly, taking breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, each one can educate you anything precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught before with better practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make much better coding behaviors transferring forward.
Documenting bugs can be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. After some time, you’ll begin to see patterns—recurring issues or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug with your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a few of the finest developers are certainly not the ones who publish ideal code, but people that continuously understand from their mistakes.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer as a result of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is big. It would make you a far more efficient, assured, and able developer. Another time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.